Data structures in Java are used to store and organize data. Data structures in Java are the backbone of any Java program. They provide a way to organize data so that it can be efficiently accessed and used.
Here is a list of data structures in Java:
Array: An array is an ordered collection of elements. It stores a fixed number of items, which are referenced by the indices, or positions, within the array.
LinkedList: A linked list is an abstract data type that represents a linear collection of data elements, or nodes, which can be accessed using sequential indices.
Stack: A stack is a container that holds its elements on top of each other so that the last element added to it will be the first one retrieved from it.
Java with Data Structures
Java is a high-level programming language that is used to build applications. It has many data structures, such as lists, binary trees, linked lists, and so on.
Java has many algorithms that can be used to sort and search data in these data structures. For example, it has sorting algorithms like quick sort and merges sort. It also has searching algorithms like a linear search and binary search.
The Java language has a rich set of fundamental data types that are used for storing values and calculations on them. These are byte, short, int, long, and char data types. There is also a boolean data type which has only two values – true or false – and a float or double data type which can store decimal numbers with around 15 digits after the decimal point of program.

Linked list program in Java
A linked list is a linear data structure which includes a group of nodes. These nodes contain data and have pointers to the next node in the list.
Linked lists are more flexible than arrays, but they are also slower because they require more processing time for insertion and deletion. A linked list is also not as space-efficient as an array.
The following example shows how to use Java code to create a linked list with three nodes:
The following example shows how to use Java code to create a linked list with three nodes:
public class LinkedList { private Node firstNode; public void addFirst(int data) { firstNode = new Node(); firstNode.data = data; firstNode.next = null; } public void print() { System.out.println(“first node: ” + firstNode); } }
Implementing a linked list in Java
Linked lists are one of the most common data structures used to store a sequence of items in computer science. It is an excellent way to store a list of objects where each object has a different type. This article will cover the basics of linked lists and how they can be implemented in Java.
In this article, we will discuss what a linked list is, how to implement one in Java, and some of its possible uses for it. We will also discuss the benefits and drawbacks of using this data structure.
What are Data Structures in C
Data structures are important in programming because they store and organize data. They can be used to solve problems that involve sorting, searching, and storing. The most common data structures are the list, the linked list, the array, the stack, and the queue.
Benefits of Data Structures
- Data Structures make to store the information on the hard disk.
- Data Structures provide abstraction and reusability.
- It is very necessary to create and design efficient algorithms.
- A large amount of data manipulation is easier.
- The relevant choice of ADT (Abstract Data Type) makes the program more efficient.
Types of Data Structures
- Primitive Data Structures
- Non-Primitive Data Structures
Primitive Data Structures
Primitive Data Structures can be directly operated by machine instructions. Similarly, Primitive Data Structures are built-in and also defined by the programming languages. Therefore some of the Primitive Data Structures’ examples are – real, character, pointer, integer, etc.
The Primitive Data Structures can only contain the values that are provided by the programmer. Some examples of Primitive Data structures are explained as follows-
Integer– The Integers are used to show only numeric data. Similarly, The integer stores all types of numeric data whether they are negative or positive.
Float– The Float is normally used to represent the fractional number. Similarly, the numbers with the decimal figures in the languages. Similarly, double can be used the increase the range.
Boolean– Normally, the boolean will only take two values that are TRUE or FALSE. Similarly, the Boolean is used for conditional testing.
Character– Character is a data type that is used to store a single value. Similarly, the value might be of upper case or lower case like “A” or “a”.

Non-Primitive Data Structures
Non – Primitive Data Structures is the complex data structure. Similarly, this is derived from Primitive data structures. Examples of Non – Primitive Data Structures are – trees, stacks, graphs, lists, etc.
Non-Primitive Data Structures are further divided into 2 categories –
- Linear Data Structures
- Non-Linear Data Structures
Linear Data Structures –
Linear Data Structures contain elements that are arranged in a sequential manner where every element is connected to its previous as well as next elements. Some of the examples of Linear Data Structure are explained as follows –
Arrays – An array in Data Structures consists of similar data elements at the connecting memory location. Similarly, it is the simplest data structure where each data element is accessed directly by only using its index number.
Stack – Stack is a type of Data Structure that follows a specific order to where its operation is being done. Similarly, the order will be LIFO ( Last in First Order ) or FILO (First In Last Out).

Linked List – A Linked List is a type of Linear Data structure that is normally used for maintaining a list like – the structure of a memory in the computer. Therefore, we can say that this is a group of nodes & each node has its adjacent node with the help of the pointers.

Queue – The Queue in Data Structure is implemented with the help of an array. Similarly, we can say that the stack data structure and the queue is most similar to each other. Therefore, the only difference between them is the elements in the queue are added and removed from the front and the rear at the end of the array.

Non-Linear Data Structures –
In the Non-Linear Data Structures, the data types are not stored sequentially. Therefore, we can say that in the Non-Linear Data Structures the data types are stored in random order. For Example, Trees & graphs are the types of Non-Linear Data Structures.
Trees – Trees are defined as the set of nodes and are a multilevel data structure. Similarly, in the trees the topmost node is said as the root node as well as a bottom node is counted as the leaf node. Therefore, we can say that each node contains a single parent but the parent can have multiple children.
Various types of Trees in Data Structures
- General Tree
- Binary Tree
- Binary Search Tree
- AVL Tree
Graph –
A graph is an illustrated representation of the objects which are connected by a link known as edges. Similarly, the nodes which are interconnected are represented by the points which are named vertices. Similarly, the links that connect the vertices are called edge.
Various types of Graphs in Data Structures
- Finite Graph
- Infinite Graph
- Simple Graph
- Multi Graph
- Null Graph
- Complete Graph
- Regular Graph
- Vertex Labelled Graph
Classification of Data Structures
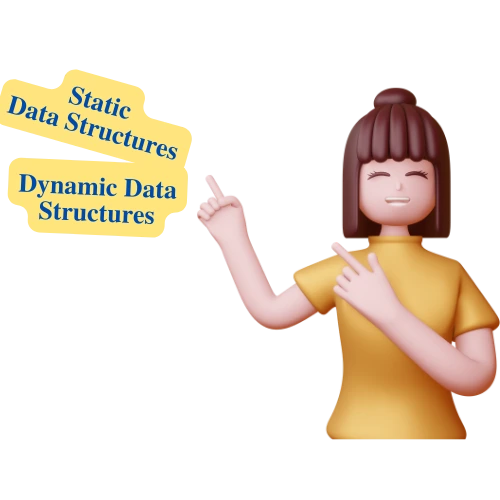
Data Structures can be classified into the 2 types that are-
- Static Data Structure
- Dynamic Data Structure
Static Data Structure – Static Data Structure is the type of structure in which the size is allocated at the time of the compilation. Therefore, the maximum size is fixed and we can’t change that.
Dynamic Data Structure – In the Dynamic Data Structures, the size is allocated at the run time. Similarly, the size is not fixed and easily changes as per the requirements.
Conclusion
With the above discussion we get to know about complete knowledge of Data Structures. Similarly, its uses as well as types. So, we can say that data structures are one of the most vital part of programming line. Therefore, whenever you decide to learn Data Structures in Jalandhar learn from experts. An GTB Computer Education, Jalandhar is one of the oldest and well-reputed computer training institute in Jalandhar. Similarly, we are in the field of last 22+ years and providing quality education to our student.

Our students are placed in many multinational companies like – TCS, WIPRO, Microsoft, etc. Therefore, whenever you want to learn Data Structures learn from experts.
For more details regarding Data Structures please visit our official website – Data Structure Training in Jalandhar – GTB Computer Education (gtbinstitute.com).